
导入依赖
导入Redis的starter
场景依赖:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-redis</artifactId> </dependency>
<dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-pool2</artifactId> </dependency>
<dependency> <groupId>redis.clients</groupId> <artifactId>jedis</artifactId> </dependency>
|
Redis相关的组件都由 RedisAutoConfiguration 完成配置和注入:
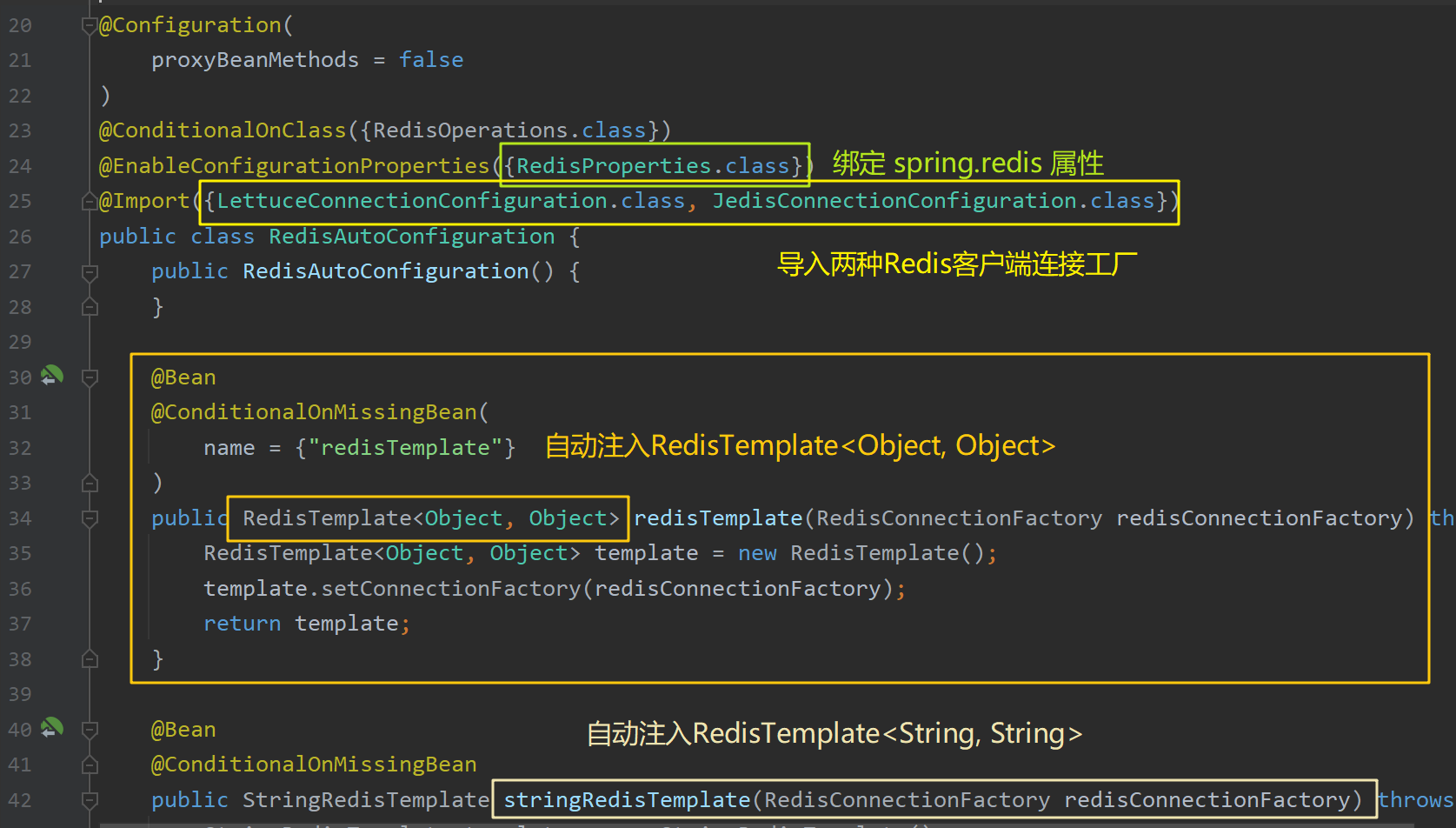
其绑定了 spring.redis
属性,并且向容器中注入了:
RedisTemplate<Object, Object>
StringRedisTemplate
,其 key-value 都是String类型
提供了两种 Redis 客户端:
- Lettuce:默认,基于Netty框架的客户端,线程安全,性能较好。
- Jedis
配置文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| spring: redis: host: 192.168.1.203 port: 6379 password: database: 0 client-type: lettuce timeout: 1800000 lettuce: pool: max-active: 10 max-wait: -1 min-idle: 5 min-idle: 0
|
Redis 配置类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
| @EnableCaching @Configuration public class RedisConfig extends CachingConfigurerSupport {
@Bean public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory factory) { RedisTemplate<String, Object> template = new RedisTemplate<>(); RedisSerializer<String> redisSerializer = new StringRedisSerializer(); Jackson2JsonRedisSerializer jackson2JsonRedisSerializer = new Jackson2JsonRedisSerializer(Object.class); ObjectMapper om = new ObjectMapper(); om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY); om.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL); jackson2JsonRedisSerializer.setObjectMapper(om); template.setConnectionFactory(factory); template.setKeySerializer(redisSerializer); template.setValueSerializer(jackson2JsonRedisSerializer); template.setHashValueSerializer(jackson2JsonRedisSerializer); return template; }
@Bean public CacheManager cacheManager(RedisConnectionFactory factory) { RedisSerializer<String> redisSerializer = new StringRedisSerializer(); Jackson2JsonRedisSerializer jackson2JsonRedisSerializer = new Jackson2JsonRedisSerializer(Object.class); ObjectMapper om = new ObjectMapper(); om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY); om.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL); jackson2JsonRedisSerializer.setObjectMapper(om); RedisCacheConfiguration config = RedisCacheConfiguration.defaultCacheConfig() .entryTtl(Duration.ofSeconds(600)) .serializeKeysWith(RedisSerializationContext.SerializationPair.fromSerializer(redisSerializer)) .serializeValuesWith(RedisSerializationContext.SerializationPair.fromSerializer(jackson2JsonRedisSerializer)) .disableCachingNullValues(); RedisCacheManager cacheManager = RedisCacheManager.builder(factory) .cacheDefaults(config) .build(); return cacheManager; } }
|
业务类测试
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| @RestController @RequestMapping("/redisTest") public class RedisTestController { @Autowired private RedisTemplate redisTemplate;
@Autowired private StringRedisTemplate redisTemplate;
@Autowired private RedisConnectionFactory redisConnectionFactory;
@GetMapping public String testRedis01() { redisTemplate.opsForValue().set("name","lucy");
String name = (String)redisTemplate.opsForValue().get("name"); return name; }
@GetMapping void testRedis02(){ ValueOperations<String, String> operations = redisTemplate.opsForValue(); operations.set("hello","world"); String hello = operations.get("hello");
System.out.println(hello); System.out.println(redisConnectionFactory.getClass()); } }
|
统计 URL 访问次数
自定义拦截器统计URL的访问次数
1 2 3 4 5 6 7 8 9 10 11 12 13
| @Component public class RedisUrlCountInterceptor implements HandlerInterceptor { @Autowired StringRedisTemplate redisTemplate;
@Override public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception { String uri = request.getRequestURI(); redisTemplate.opsForValue().increment(uri); return true; } }
|
注册URL统计拦截器:
1 2 3 4 5 6 7 8 9 10 11 12 13
| @Configuration public class AdminWebConfig implements WebMvcConfigurer{ @Autowired RedisUrlCountInterceptor redisUrlCountInterceptor;
@Override public void addInterceptors(InterceptorRegistry registry) { registry.addInterceptor(redisUrlCountInterceptor) .addPathPatterns("/**") .excludePathPatterns("/","/login","/css/**","/fonts/**","/images/**", "/js/**","/aa/**"); } }
|
调用Redis内的统计数据:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| @Slf4j @Controller public class IndexController { @Autowired StringRedisTemplate redisTemplate;
@GetMapping("/main.html") public String mainPage(HttpSession session,Model model){ log.info("当前方法是:{}","mainPage");
ValueOperations<String, String> opsForValue = redisTemplate.opsForValue();
String s = opsForValue.get("/main.html"); String s1 = opsForValue.get("/sql");
model.addAttribute("mainCount",s); model.addAttribute("sqlCount",s1);
return "main"; } }
|